Middlewares are useful features in Web development that helps manage what happens when a user makes a request to your server. In this blog, we’ll focus on how to create simple middleware for handling requests in standard routing. We'll also explore practical examples so you can test it yourself.
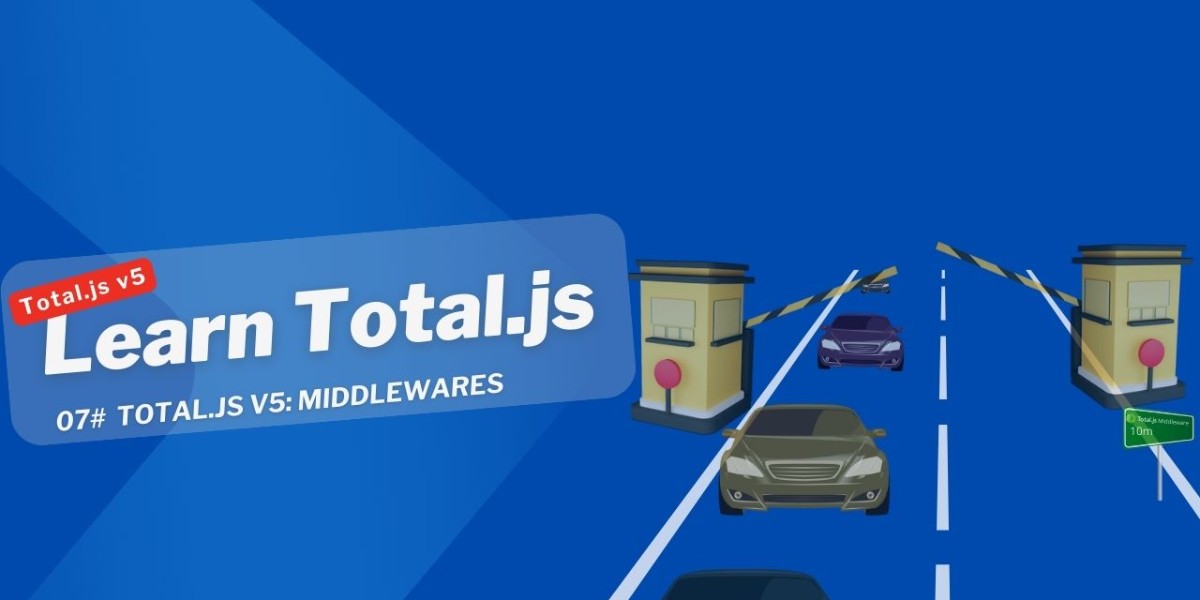
Total.js V5: Middlewares
Middlewares are useful features in Web development that helps manage what happens when a user makes a request to your server. It acts like a checkpoint, where you can inspect the request, modify it, or stop it if needed, before it reaches the main logic (controller or module).
In this blog, we’ll focus on how to create simple middleware for handling requests in standard routing. We'll also explore practical examples so you can test it yourself.
1. What is Middleware?
Think of middleware as a gatekeeper that controls access to your routes. For example, before someone can access a secure page, middleware can check if they are logged in. If not, it blocks them with an error message.
Total.js allows you to create middleware for various types of requests:
- Standard (dynamic) routes: Used for API calls or normal page requests.
- Static files: Middleware for files like images, CSS, or JavaScript.
- WebSocket: Middleware for WebSocket connections (real-time messaging).
For now, we’ll focus on middleware for standard routes.
2. How to Create and Use Middleware
Step 1: Create middleware
In Total.js, you can create middleware by using the MIDDLEWARE()
function. Here’s a simple example:
This middleware checks if a user is logged in ($.user). If yes, it lets the request continue using next(). If not, it stops the request and sends a 401 Unauthorized error.
Step 2: Applying middleware to a route
Once you have created middleware, you can apply it to a specific route. For example:
In this example:
The route /api/users/ is protected by the auth middleware.
If a user tries to access it without being logged in, they’ll receive an Unauthorized error.
Organizing Middleware
It’s a good idea to store all your middleware in a separate folder to keep things organized:
Then, add your definitions
files inside this folder, like auth.js
.
Practical example: protecting a route with Bearer Token
In this example, we’ll set up middleware that checks for a specific Bearer token in the headers of a request. If the correct token is provided, the user can access the route. Otherwise, they receive a 401 Unauthorized response.
Step 1: Create Middleware File
Inside the difinitions
folder, create a file named auth.js
:
This middleware checks the Authorization header for a Bearer token. In this case, we are expecting the token to be Bearer mysecrettoken.
Step 2: Applying middlewares to routes
Now, in your main file (e.g., index.js), add two routes:
One that requires the correct Bearer token
to access.
One that returns a 401
Unauthorized message if the token is missing or incorrect.
Step 3: Testing
Testing with Postman:
Run Your Server: Start your Total.js server.
node index.js
Set Up the Request in Postman:
Method: GET
URL: http://localhost:8000/api/welcome/
Headers:
- Key:
Authorization
- Value:
Bearer mysecrettoken
Send the Request: If you provide the correct token (Bearer mysecrettoken), you’ll get the following response:
Without the Token: If you don't include the Authorization header or provide the wrong token, you'll get an error:
The middleware will block access and redirect the user to the /api/unauthorized/
route, which returns the 401
Unauthorized message.
How the Middleware Works
The middleware looks for the Authorization header in each incoming request.
If the header contains the correct Bearer token ( Bearer mysecrettoken
), the request is allowed to proceed to the next step.
If the token is missing or incorrect, the middleware blocks the request and sends a 401
Unauthorized response.
This approach allows you to secure your API
routes easily with a token-based
authentication system. You can use this technique to verify users, protect sensitive routes, or manage access control.
Conclusion
Middleware is a simple yet powerful tool in Total.js. By using it, you can control access to your routes, validate requests, and manage other actions before they reach the core of your application. With just a few lines of code, you can secure your app’s routes and prevent unauthorized access.
Now that you understand the basics, try applying middleware to different routes in your project and experiment with more complex logic!
Other posts from Total.js Platform
- 2025-01-01December report 2024
- 2024-12-22Merry Christmas and a Happy New Year 2025!
- 2024-12-10Total.js UI Builder: How to upload files?
- 2024-12-04Performance Testing: Total.js vs. Koa
- 2024-12-03Total.js UI Builder: Creating a Form
- 2024-12-02November report 2024
- 2024-11-26Total.js V5: Schemas and Actions
- 2024-11-25QueryBuilder in Action Part 1
- 2024-11-13Benchmarking Node.js Frameworks: selecting your framework for 2025!
- 2024-11-01October report 2024