This blog covers the essentials of client-side routing in Total.js UI, explaining how to dynamically load content without reloading the entire page.
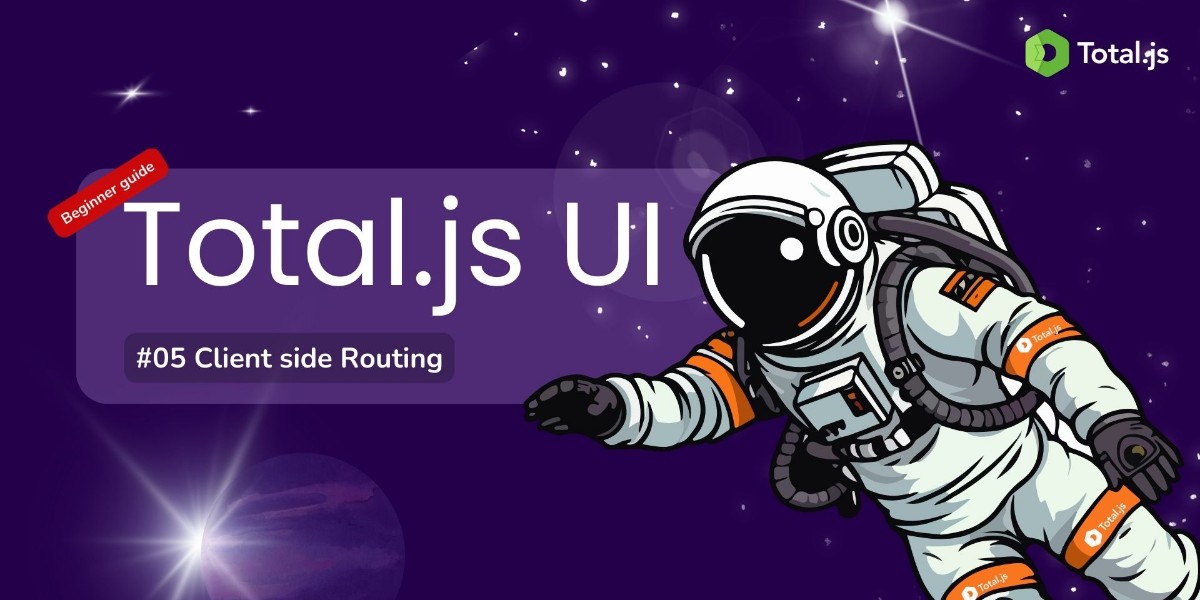
Beginner Guide to Total.js UI: #05 Client-side routing
For those who have been following along, you should now have a solid understanding of data binding, which allows you to create dynamic and interactive user interfaces in Total.js UI . With that knowledge in hand, you’re ready to move on to another core concept: client-side routing.
Client side Routing is what makes your web applications dynamic, enabling smooth transitions between pages or views without reloading the entire page. Whether you're familiar with routing from other front-end frameworks like React or Vue, or you're new to the idea, mastering routing in Total.js UI will empower you to create fluid and responsive user experiences.
In this post, we’ll explore how client-side routing works in Total.js UI, focusing on the ROUTE
method and NAV.clientside()
function. By the end of this post, you'll have a strong foundation in routing, preparing you for more advanced topics in future posts.
Let’s dive deeper into how these methods work and how to use them to create dynamic client-side routing for your application.
Table of Contents
- Prerequisites
- Understanding Client-Side Routing
- Understanding Client-Side Navigation
- Practical Example: Building a Simple Client-Side Routing Application
- Conclusion
1. Prerequisites
Before diving into client-side routing, make sure you’ve reviewed our previous post on Data Binding in Total.js UI to get familiar with the basics of Total.js UI. Here’s a quick list of essentials:
- HTML & JavaScript: Basic understanding to work with page components and route logic.
- jQuery: Familiarity is helpful, though not mandatory.
- Modern Browser & Code Editor: Use Chrome, Firefox, or Edge with editors like VS Code, Sublime Text, or Atom.
1.1 Setting Up the Project
For client-side routing to work properly, we need a server-side route to serve the single-page application (SPA). We will use the Total.js framework (server-side) to serve the client-side HTML on a specific port.
You can also skip the manual setup by using the Total.js SPA Starter Template built with Total.js 4. This template includes a pre-configured structure for an SPA project, making it easier to get started.
Step 1: Create a New Project Directory
If you want to set up your project manually, create a folder for your project and navigate into it:
Step 2: Initialize the Project
Initialize a new Node.js project with npm
:
Install the Total.js framework (version 5):
Step 3: Set Up the Server
Create the entry point for the server by creating the index.js
file:
Add the following code to index.js
to set up the Total.js server:
This starts the Total.js server on port 3000
.
Step 4: Create a Controller
Controllers define the routes and logic for serving pages. Create a controllers
directory and a default controller:
Add the following to controllers/default.js
to serve your HTML:
This code sets up a GET route for /
that serves the index.html
file.
Step 5: Set Up Views
Views are where the HTML content is placed. Create a views
directory and an index.html
file:
Add the following code to views/index.html
, which will be the entry point for your SPA:
This serves a simple HTML page using Total.js on the server.
Step 6: Start the Server
Finally, run the following command to start the server:
Now, visit http://localhost:3000
to see your application running. At this point, we are serving a single-page HTML file, and from here, we can start working with client-side routing using Total.js UI.
If you'd prefer not to set up the project manually, you can quickly get started by using the Total.js SPA Starter Template, which provides a pre-built structure for building a single-page application.
With everything in place, you're ready to implement client-side routing using ROUTE
and NAV.clientside()
in Total.js UI!
Understanding Client-Side Routing
Client-side routing is essential for building modern single-page applications (SPAs), where users can navigate between different sections of the application without reloading the entire page. In Total.js UI, client-side routing dynamically loads content based on the URL path, providing a smooth, seamless user experience.
2.1 What is Client-Side Routing?
In a traditional web application, navigation involves sending a new request to the server for every new page, causing the entire page to reload. However, SPAs handle navigation differently—when users click on links or interact with navigation elements, the browser intercepts these events. The URL is updated, but instead of reloading the page, content is dynamically loaded within the same page.
This dynamic behavior is made possible by client-side routing, which listens for changes in the URL and loads appropriate views or components without disrupting the user experience. Total.js UI's routing framework enables this by using the ROUTE()
function to define URL patterns and the NAV.clientside()
method to manage transitions between different views.
Key Concept:
- SPA (Single Page Application): A type of web application that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from the server. This reduces load times and improves user experience.
2.2 How Does Total.js UI Handle Routing?
In Total.js UI, client-side routing is managed through the NAV.clientside()
function. This function is responsible for listening to changes in the browser's URL, intercepting link clicks, and triggering the appropriate actions or views based on the current URL path. The result is a faster and more fluid user experience, as only the relevant parts of the page are updated.
Example:
In this example, when the user navigates to /about/
, the ROUTE()
function triggers an action that updates the common.page
value to 'about'
, which dynamically loads the "About Us" content without a full page reload.
Core Functions:
ROUTE(path, handler)
: This function defines specific URL paths and associates them with actions or view updates. In this case, it triggers the content associated with the/about
route.
Read more about the ROUTE()
function in the Total.js documentation.
NAV.clientside()
: This function initializes client-side routing, listens for URL changes, and loads the appropriate content based on the current route. By callingNAV.clientside()
, you can ensure that all navigation happens within the browser without refreshing the page.
2.3 Benefits of Client-Side Routing in Total.js UI
- Faster Navigation: Since the browser doesn’t have to reload the entire page, transitions between views are near-instantaneous.
- Enhanced User Experience: Users experience smoother, uninterrupted interactions as content is loaded dynamically.
- Simplified Development: Total.js UI's
ROUTE()
andNAV.clientside()
functions abstract away the complexities of routing, making it easier to build dynamic, responsive SPAs.
2.4 Example of Setting Up Client-Side Routing
Here’s a simple example of setting up client-side routing for an SPA using Total.js UI:
In this setup:
- The root path
/
loads the home page. - Navigating to
/about
dynamically loads the "About Us" section. - Navigating to
/contact
dynamically loads the contact page.
The NAV.clientside()
function ensures that these routes are managed on the client side without refreshing the entire page.
2.5 Dynamic Routing
Dynamic routing allows your application to handle URLs with variable segments, enabling you to create more flexible and interactive routes. This is particularly useful for handling user-specific or resource-specific URLs, such as viewing a profile page for a particular user or accessing details for a specific item.
In Total.js UI, dynamic routes are defined by placing placeholders within curly braces {}
inside the route path. These placeholders capture the dynamic part of the URL, allowing you to process parameters like user IDs, product codes, or any other variable data directly within the route handler.
Example of Dynamic Routing:
In this example, {id}
represents a dynamic segment of the URL. If the user navigates to /user/123
, the id
variable will be assigned the value 123
. This allows you to retrieve data specific to the user with ID 123, such as loading their profile page or fetching data from the server.
How It Works:
- The placeholder
{id}
captures the dynamic part of the URL. - When a user navigates to a URL matching the route pattern (e.g.,
/user/123
), the captured value (123
) is passed to the route handler as a function argument.
This approach allows you to create dynamic, data-driven pages that are tailored to individual users or resources based on URL parameters.
2.6 Combining Static and Dynamic Routes
Dynamic routes can be combined with static parts of the URL to further structure your application. For example, you might want to create a route for displaying specific products based on their ID, while keeping other parts of the URL static.
Example:
In this case, /product/{productId}
is a dynamic route where {productId}
can be any identifier for a product. When a user navigates to /product/456
, the productId
variable will be set to 456
, and the product-details
page will be dynamically loaded.
Key Points of Dynamic Routing:
- Flexible URLs: Allows the creation of routes that can handle a wide range of URL patterns.
- Data-Driven: Captures dynamic parts of the URL, which can be used to fetch and display resource-specific data.
- Efficient Page Loading: Dynamically loads different views or data without requiring a full page reload.
By leveraging dynamic routing, you can create highly interactive SPAs in Total.js UI, offering users personalized experiences based on URL parameters.
Query Parameters
Query parameters add additional information to the URL, which can be useful for searches or filters.
Example of Query Parameters:
If the URL is /search/?q=totaljs
, the parameter q
will be totaljs
.
2.7 Controllers in Client-Side Routing
In Total.js UI, controllers handle the logic for specific routes. When a route is matched, its corresponding controller (or route handler) is executed, determining what action should be performed. The controller is responsible for managing views, interacting with models, and updating the application state based on user actions or URL changes.
When you define a route using ROUTE()
, the handler function associated with the route acts as the controller for that route.
Example:
In this example, the route /about/
is associated with a function that acts as the controller. This function is responsible for handling the logic when a user navigates to the "About Us" page. The SET('common.page', 'about')
updates the current view by setting the common.page
property to 'about'
, which dynamically loads the "About Us" content.
The this
Keyword in Controllers
When the route controller is triggered, the this
keyword provides access to a context object containing valuable information about the current route, user environment, and more. By logging this
in the console, you can inspect the properties available to the controller.
Example Console Output for this
:
Key Properties of the Controller Context (this
):
version
: Indicates the version of Total.js UI being used.url
: The current route path, such as/about/
.routes
: An array of all defined routes, including the current route with details like the URL path, priority, and route count.history
: Tracks the navigation history, useful for understanding the user’s movement between pages (e.g.,["/", "/about/"]
).ua
: Contains user agent information, such as the operating system, browser, and device type (e.g.,"os": "Mac", "browser": "Chrome", "device": "desktop"
).query
andparams
: Store query parameters and dynamic route parameters, respectively. These can be accessed directly in the route handler to further customize page content.
Using this
for Route Debugging
The context provided by this
is useful for debugging and understanding how the route is being processed. For example, you can examine the current route URL, track how many times the route has been visited (count
), and check whether the user is navigating back or forward in history (isback
, isforward
).
Example Use of this
:
In this example, the id
variable holds the dynamic URL segment (e.g., /user/123
), and the this
object provides additional information about the route and environment. You can use this data to enhance the logic within your route handler.
Benefits of Controllers in Client-Side Routing:
- Centralized Logic: Controllers manage all the logic related to a route, making it easy to update and maintain.
- Dynamic Content: Controllers can respond to dynamic URLs and query parameters, ensuring the right data is loaded for each page.
- Contextual Information: The
this
object provides a rich set of information about the current state of the application, including history, user agent, and URL parameters.
By using controllers in client-side routing, you ensure your Total.js UI application remains organized, flexible, and easy to maintain, especially as the number of routes and views grows.
2.8 Client-Side Navigation in Total.js UI
Client-side navigation is crucial in Single Page Applications (SPAs) because it allows users to navigate between different sections of the application without triggering a full page reload. This results in faster load times and a smoother user experience. In Total.js UI, client-side navigation is managed using the NAV.clientside()
method, which intercepts link clicks and routes users to the appropriate view dynamically.
Route Binding with NAV.clientside()
The NAV.clientside()
method binds the client-side navigation system to specific links, allowing seamless navigation within your application without requiring server round-trips.
Example of Route Binding:
In this example, the navigation system is bound to all anchor (<a>
) elements that have the class routing
. Whenever the user clicks on any of these links, the NAV.clientside()
method intercepts the event and updates the view dynamically, without reloading the entire page.
Dynamic Navigation Controls
Total.js UI offers several methods to manipulate navigation programmatically, enabling you to control page transitions manually.
NAV.back()
: This method navigates back to the previous page in the browser’s history stack.NAV.forward()
: Moves to the next page in the history stack (if the user previously navigated back).NAV.refresh()
: Reloads the current view, useful for reloading content without changing the URL or reloading the entire application.
Examples of Navigation Controls:
These navigation controls provide flexibility when managing navigation flows, especially in complex SPAs where users may navigate across multiple views frequently.
Use Cases for Client-Side Navigation:
- Improving Performance: By avoiding full page reloads, client-side navigation makes the application feel faster and more responsive.
- Enhanced User Experience: Pages transition smoothly without disrupting the user flow, resulting in a more polished experience.
- Custom Navigation Controls: You can build custom navigation behavior, such as multi-step forms, or control how users move between specific sections of the application based on their actions.
Combining NAV.clientside()
with Other Features
The power of client-side navigation in Total.js UI comes from its ability to integrate with other features like controllers, dynamic routing, and data binding. For example, when users navigate between views, you can automatically load new data or trigger specific actions based on the URL path, without reloading the entire page.
By leveraging NAV.clientside()
along with the navigation controls like NAV.back()
, NAV.forward()
, and NAV.refresh()
, you can build complex and interactive SPAs with minimal effort.
Conclusion
Client-side routing is a key aspect of building modern SPAs, enabling dynamic content updates without full page reloads. Total.js UI provides a straightforward and powerful framework for managing client-side navigation using the ROUTE
function and NAV.clientside()
method. By defining static and dynamic routes, handling query parameters, and leveraging navigation controls, you can create seamless, responsive web applications that deliver a smooth user experience.
In the next post, we'll dive into a practical example of client-side routing in Total.js UI, where we'll build a simple application to demonstrate how to set up and manage routes, handle dynamic parameters, and control navigation—all in a hands-on tutorial. Stay tuned!
Other posts from Total.js Platform
- 2025-04-14Beekeeping - recording damage caused by a brown bear
- 2025-04-11j-Importer - Total.js
- 2025-04-10The Thurzo House Museum
- 2025-04-02March report 2025
- 2025-03-28j-DataGrid - Total.js UI component part 2
- 2025-03-20j-DataGrid - Total.js part 1
- 2025-03-13j-Directory - jComponent
- 2025-03-05j-Input UI component part 2
- 2025-03-01February report 2025
- 2025-02-03January report 2025